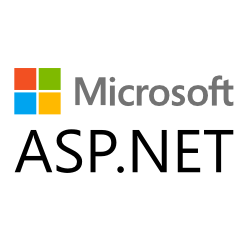
One of the common requirements when using ASP.NET Identity, is adding new properties to the User entity. For example let’s say, we want to add a Code
property to IdentityUser
entity to store personnel code for the user.
In this post I’ll share a step by step guide which is inspired by my answer to this question in stackoverflow about adding new property to Identity user.
By following all the steps below, you can add a Code
property to IdentityUser
. Here is all steps to add a new property to user:
- Create an ASP.NET Web Application
- Make sure you select MVC and the Authentication is Individual User Accounts
- Go to Models folder → Open IdentityModels.cs → ApplicationUser class and add the property:
public string Code { get; set; }
- Build the project
-
Go to TOOLS menu → Nuget Package Manager → click Package Manager Console
-
Type
Enable-Migrations
and press Enter and wait until the task get completed. You will see a response which says:Checking if the context targets an existing database... Code First Migrations enabled for project WebApplication1.
- Type
Add-Migration "Code"
and press Enter and wait until the task get completed. You will see a response which says:Scaffolding migration 'Code'. The Designer Code for this migration file includes a snapshot of your current Code First model. This snapshot is used to calculate the changes to your model when you scaffold the next migration. If you make additional changes to your model that you want to include in this migration, then you can re-scaffold it by running 'Add-Migration Code' again.
- Type
Update-Database
and press Enter and wait until the task get completed. You will see a response which says:Specify the '-Verbose' flag to view the SQL statements being applied to the target database. Applying explicit migrations: [201611132135242_Code]. Applying explicit migration: 201611132135242_Code. Running Seed method.
At this step if you refresh SQL Server Object Explorer and go to database and see tables, under
dbo.AspNetUsers
under columns, you will see theCode
field. If you didn’t know which database or even which server you should look for, open Web.Config file and take a look at connection string which is something like this:<add name="DefaultConnection" connectionString="Data Source=(LocalDb)\v11.0;AttachDbFilename=|DataDirectory|\aspnet-WebApplication1-20161114125903.mdf;Initial Catalog=aspnet-WebApplication1-20161114125903;Integrated Security=True" providerName="System.Data.SqlClient" />
You can see data source (which is sql server instance) and something .mdf which is database name.
-
Go to Models folder → Open AccountViewModels.cs file → RegisterViewModel class and add this property: (In APIv2 with EF6, you can add the below line in Models folder → AccountBindingModels file → RegisterBindingModel class)
public string Code { get; set; }
- Go to Views folder → Account folder → Open Register.cshtml file and add this code near other fields, for example below password:
<div class="form-group"> @Html.LabelFor(m => m.Code, new { @class = "col-md-2 control-label" }) <div class="col-md-10"> @Html.TextBoxFor(m => m.Code, new { @class = "form-control" }) </div> </div>
- Go to Controllers folder → Open AccountController.cs file → in http post Register action, change the line which creates user to this:
var user = new ApplicationUser { UserName = model.Email, Email = model.Email, Code= model.Code };
- Run project and go to
/Account/Register
url and register a new user. After registering the user, if you go to database again and View Data of dbo.AspNetUsers table, you will see the code has been saved.
Download
You can clone or download a working example here:
hello,
how can i retrive the custom property afetr login?
Thanks.