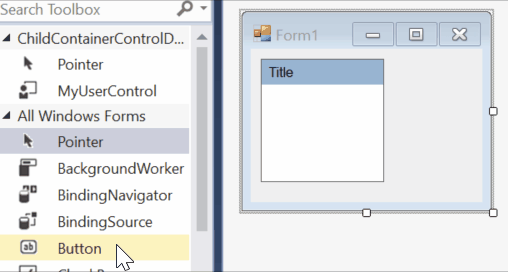
Sometimes you want to create a composite user control and allow users to interact with the user control at designer when they drop an instance of your user control on a form. For example, let’s say we are going to create a UserControl
containing a title panel and a contents panel with following requirements:
- Users should be able to drop controls on the contents panel at design time.
- Users should not be able to drop controls on title panel.
- Users should not be able to select title panel or change its properties.
- Users should be able to select contents panel.
- Users should be able to change properties of contents panel.
- Users should not be able to move or delete contents panel.
In this post I’ll show how you can create a composite control (UserControl) and let the user to interact with a child control of your user control:
Prevent from drop item of forms:
Prevent from drop items from toolbox:
To satisfy above requirement, you need to create custom designers for the user control and for the contents panel.
Create the UserControl Create a user control having the following layout:
Here is the code for the user control:
[Designer(typeof(MyUserControlDesigner))]
public partial class MyUserControl : UserControl
{
public MyUserControl()
{
InitializeComponent();
TypeDescriptor.AddAttributes(this.contentsPanel,
new DesignerAttribute(typeof(MyPanelDesigner)));
}
public string Title
{
get { return titleLabel.Text; }
set { titleLabel.Text = value; }
}
[DesignerSerializationVisibility(DesignerSerializationVisibility.Content)]
public Panel ContentsPanel
{
get { return contentsPanel; }
}
}
In above code:
- We have registered the designer of the user control
- Also we registered the designer of the contents panel in constructor pf our user control. Since contents panel is a panel, it will use
PanelDesigner
automatically, but here by registering a newDesignerAttribute
pointing to our custom panel designer, we will tell the designer to use our custom panel designer. The reason that we put it into constructor is because we want to be able to usePanelDesigner
in design time of our user control, but we want to use our custom designer when we drop an instance of user control on the form. - We expose the contents panel as a readonly property of the control and will tell the designer to serialize its contents. Later in the custom designer for our user control, we will use this property to enable the designer for the content panel:
Create designer for user control
We need to create a custom designer for the user control. Here are the main responsibilities for the designer for our user control:
- Enable designer for contents panel
- Prevent users from dropping contents into user control. (The only part of user control which accepts dropping controls, is contents panel)
Here is the code for user control designer:
public class MyUserControlDesigner : ParentControlDesigner
{
public override void Initialize(IComponent component)
{
base.Initialize(component);
var contentsPanel = ((MyUserControl)this.Control).ContentsPanel;
this.EnableDesignMode(contentsPanel, "ContentsPanel");
}
public override bool CanParent(Control control)
{
return false;
}
protected override void OnDragOver(DragEventArgs de)
{
de.Effect = DragDropEffects.None;
}
protected override IComponent[] CreateToolCore(ToolboxItem tool,
int x, int y, int width, int height, bool hasLocation, bool hasSize)
{
return null;
}
}
In above code:
- By calling
EnableDesignMode
method, we have enabled the contents panel at design time to allow user interaction with the contents panel. - We override
CanParent
method to prevent user from dragging control of the form into the user control. - We override
CreateToolCore
to prevent users from dropping controls from toolbox on the user control. - We override
OnDragOver
to show suitable mouse icon that shows dropping is not allowed on user control.
Create designer for contents panel
We need to create a custom designer for the contents panel. Here are the main responsibilities for the designer of our contents panel:
- Prevent contents panel from moving, sizing and deleting.
- Remove some properties like
Dock
,Visible
,Location
,Size
, etc. - Remove Dock in Parent Container and Undock in Parent Container.
Here is the code for contents panel designer:
public class MyPanelDesigner : ParentControlDesigner
{
public override SelectionRules SelectionRules
{
get
{
SelectionRules selectionRules = base.SelectionRules;
selectionRules &= ~SelectionRules.AllSizeable;
return selectionRules;
}
}
protected override void PostFilterAttributes(IDictionary attributes)
{
base.PostFilterAttributes(attributes);
attributes[typeof(DockingAttribute)] = new DockingAttribute(DockingBehavior.Never);
}
protected override void PostFilterProperties(IDictionary properties)
{
base.PostFilterProperties(properties);
var propertiesToRemove = new string[] {
"Dock", "Anchor",
"Size", "Location", "Width", "Height",
"MinimumSize", "MaximumSize",
"AutoSize", "AutoSizeMode",
"Visible", "Enabled",
};
foreach (var item in propertiesToRemove)
{
if (properties.Contains(item))
properties[item] = TypeDescriptor.CreateProperty(this.Component.GetType(),
(PropertyDescriptor)properties[item],
new BrowsableAttribute(false));
}
}
}
I above code:
- We override
SelectionRules
to prevent moving, sizing and deleting the contents panel. - We override
PostFilterProperties
method to remove some properties from designer. - We override
PostFilterAttributes
to remove Dock in Parent Container and Undock in Parent Container.
Download
You can clone or download the working example:
This was amazingly helpful! I would have not figured out how to do this by myself, so thanks a lot!
The only small hangup I had was understanding which namespaces I needed to import into my classes (System.Windows.Forms.Design, and System.ComponentModel ) and the additional assembly I needed to reference in my project (System.Drawing). But other that that, I appreciate your clear explanations about how this is all working. So, thanks again!
This is the best guide I have seen on this UserControl topic! Thank your for taking the time to document this process and make to clear and easy to follow.
Great! Thanks
Quick question … first, thanks for the help and code! Current issue..I had my user control working perfectly … but then wanted to remove the panel so that my combobox and label where directly on the UC instead of on the panel. This caused a strange problem which I can’t seem to figure out .. I can still select and resize the individual controls (combobox, etc) but when I try to move them, even though I get the little 4-arrows move icon .. when I move it , it snaps back to original location. Nothing is docked, the only thing that changed was that the controls are now on the UC directly and not on a panel.
Any help greatly appreciated.
– Karlton
@Karlton, I just checked the GitHub project again and everything works well. You may want to download/clone the project and start the customization based on that.
Hi there
Thanks for that.
Under .Net 4.7.2 it is perfect.
However, if you try it under .Net Core 3 the behaviour is not quite right. Child controls can be placed on the title bar. And when you reopen your form, child controls are no longer visible (but they are still there and can be clicked on and set to Bring to Front to be visible). You also can’t see the controls when running the app.
I think the .Net Core form designer has a few bugs in it.
Works fine under .Net 4.6.2 but does not work under .Net Core 3. I tried it under both frameworks. It probably needs some tweaks under .Net Core 3.
Reza please There’s a problem with the WinForms designer. I try your solution it’s 100% and awesome. But I can’t get Forms from the current assembly.
please see here
https://stackoverflow.com/questions/58514948/user-control-property-that-shows-a-list-of-all-forms-at-design-time