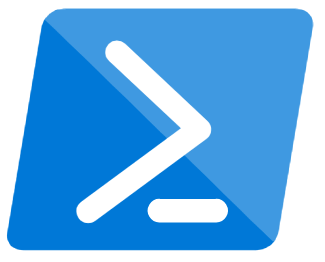
Sometimes in a PowerShell function you need to know which parameters are passed to the method. For example you may have an optional parameter and you want to know if the optional parameter is passed to method or not. As a common scenario, let’s suppose we have a function called Update-Values
accepting p1
and p2
parameters and the function should update those values in a json file if the user passed those values.
For example let’s suppose we have a json file like following:
{
"p1": "value1",
"p2": "value2"
}
And a function like following:
Function Update-Values (p1, p2) {
# If p1 is passed, update p1 in file
# If p2 is passed, update p2 in file
}
For either of those parameters, if the value is passed to the method, user wants to update the value in the file.
In most cases, I’ve seen developers compare the parameter value with null or empty to see if the parameter is passed to the function or not:
# Sometimes it's not good idea to check the parameter existence this way:
if($p1) {Write-Host "p1 exists"}
else {Write-Host "p1 not exists"}
Sometimes it works. But in some cases, the user’s intention is setting the parameter value to null or empty and his intention is to save null or empty value to file as well. If you use above method, you can not distinguish between following cases:
- User wants to just update
p1
, but he don’t want changep2
:Update-Values -p1 "something"
- User wants to just update
p1
, and want to setp2
tonull
:Update-Values -p1 "something" -p2 $null
So how can we distinguish between above cases?
How can you detect if a parameter is passed, for an optional parameter which can accept null as value?
As an option you can use $PSBoundParameters
collection to check whether passed to the method:
$PSBoundParameters.ContainsKey("paramater name")
For example having following function, we can find out whether p1 and p2 parameters are passed to the function:
Function Update-Values ($p1, $p2) {
If($PSBoundParameters.ContainsKey("p1")) {
Write-Host "p1 exists"
}
else {
Write-Host "p1 not exists"
}
If($PSBoundParameters.ContainsKey("p2")) {
Write-Host "p2 exists"
}
else {
Write-Host "p2 not exists"
}
}
Then if you run the function using the following parameter:
Update-Values -p1 "something"
As a result you will see:
p1 exists
p2 not exists