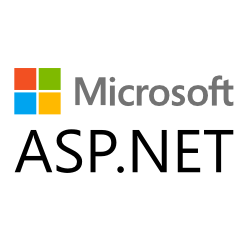
In previous post, I showed you how to add property to Identity user. Another common requirement when using ASP.NET Identity is adding new property to IdentityRole
. In this post I’ll share a step by step guide which is inspired by my answer to this question question in stackoverflow about adding new property to Identity role.
To add a new property to IdentityRole
, you can follow the following steps:
- Create an ASP.NET Web Application
- Make sure you select MVC and the Authentication is Individual User Accounts
- Go to Models folder → Open IdentityModels.cs and Create ApplicationRole class containing the custom property that you want to add:
public class ApplicationRole : IdentityRole { public string Description { get; set; } }
- Change
GenerateUserIdentityAsync
method ofApplicationUser
to accept parameter of type ofUserManager<ApplicationUser, string>
:public class ApplicationUser : IdentityUser { public async Task<ClaimsIdentity> GenerateUserIdentityAsync(UserManager<ApplicationUser, string> manager) {
- Change
ApplicationDbContext
base class and introduce all the generic parameters:public class ApplicationDbContext : IdentityDbContext<ApplicationUser, ApplicationRole, string, IdentityUserLogin, IdentityUserRole, IdentityUserClaim> { public ApplicationDbContext() : base("DefaultConnection") {
- Build the project.
-
Go to TOOLS menu → Nuget Package Manager → click Package Manager Console
-
Type
Enable-Migrations
and press Enter and wait until the task get completed. - Type
Add-Migration "ApplicationRole"
and press Enter and wait until the task get completed. - Type
Update-Database
and press Enter and wait until the task get completed. -
Go to App_Start folder → Open IdentityConfig.cs and Change the
ApplicationUserManager
class to derive fromUserManager<ApplicationUser, string>
and also change itsCreate
method to return aUserManage
aware ofApplicationRole
:public class ApplicationUserManager : UserManager<ApplicationUser, string> { public ApplicationUserManager(IUserStore<ApplicationUser, string> store) : base(store) { } public static ApplicationUserManager Create(IdentityFactoryOptions<ApplicationUserManager> options, IOwinContext context) { var manager = new ApplicationUserManager(new UserStore<ApplicationUser, ApplicationRole, string, IdentityUserLogin, IdentityUserRole, IdentityUserClaim>(context.Get<ApplicationDbContext>()));
- To manage roles, create
ApplicationRoleManager
class in the same file:public class ApplicationRoleManager : RoleManager<ApplicationRole> { public ApplicationRoleManager(IRoleStore<ApplicationRole, string> store) : base(store) { } public static ApplicationRoleManager Create( IdentityFactoryOptions<ApplicationRoleManager> options, IOwinContext context) { return new ApplicationRoleManager(new RoleStore<ApplicationRole>(context.Get<ApplicationDbContext>())); } }
- Go to App_Start folder → Open Startup.Auth.cs and add the following code to the
ConfigureAuth
method:ConfigureAuthapp.CreatePerOwinContext<ApplicationRoleManager>(ApplicationRoleManager.Create);
Now the project is ready to take advantage of the new ApplicationRole
.
It’s works and great article, thanks!
Good post, thanks.
سپاس فراوان